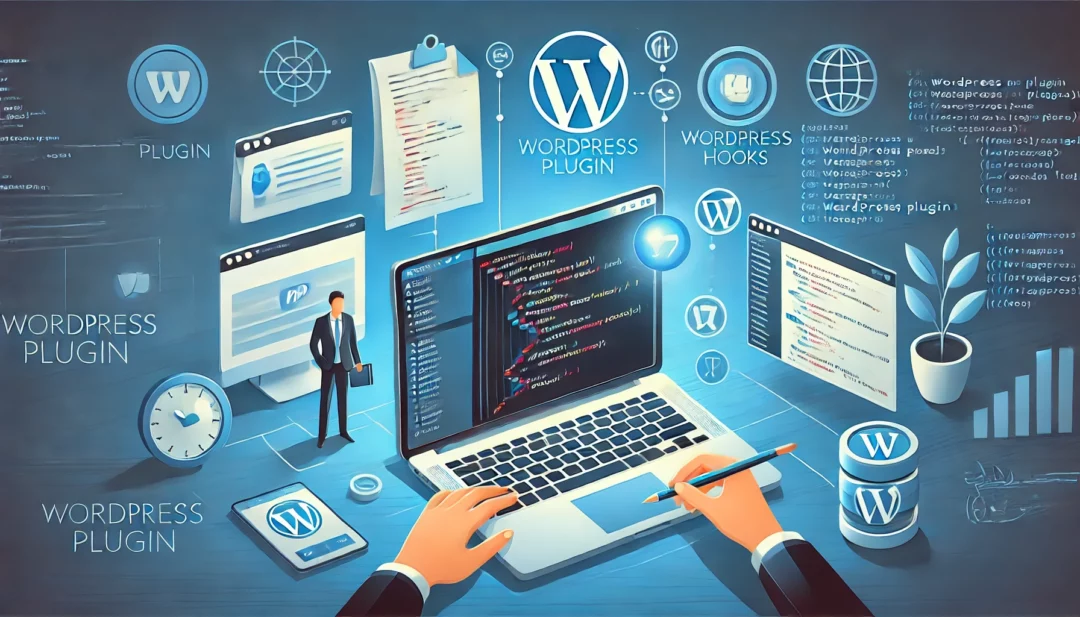
Creating your own WordPress plugin might sound daunting, but it’s simpler than you think. Whether you’re looking to add custom functionality or enhance your website’s features, building a plugin gives you full control. With just a few basic steps, you can turn your ideas into a functional plugin that integrates seamlessly with WordPress.
To get started, you’ll define your plugin’s purpose, set up the necessary files, and write the core code. From there, you’ll test your plugin for functionality and compatibility before activating it on your site. Each step is straightforward and designed to help you bring your vision to life without unnecessary complexity. By the end, you’ll have a working plugin tailored to your needs.
Understanding WordPress Plugins
WordPress plugins are essential tools for enhancing website functionality without altering core code. Learning about plugins helps you create tailored solutions that meet your unique requirements.
What Are Plugins And Why They Matter
Plugins are add-ons that expand WordPress features or integrate third-party services into your website. Each plugin contains PHP code that connects seamlessly with WordPress through hooks and filters. Examples include SEO tools, contact forms, and performance optimizers.
They matter because they let you customize your website efficiently. Instead of modifying the core CMS, you can add or remove features as needed. This modular approach keeps updates manageable and prevents conflicts with WordPress itself.
Benefits Of Creating Your Own Plugin
Creating your own plugin offers flexibility, enhanced control, and cost savings. You avoid reliance on existing plugins, and you ensure functionality aligns with your site’s goals.
- Tailored Features: Solve unique problems specific to your site. For example, you could create custom post types or automate backend tasks.
- Improved Security: Minimize third-party vulnerabilities by developing controlled solutions.
- Learning Opportunities: Develop skills in PHP scripting, WordPress API, and development best practices.
- Scalability: Create expandable modules that evolve with your website’s needs. For instance, start with basic functionality, then add advanced features later.
Developing plugins can optimize performance, reduce compatibility issues, and provide a competitive edge.
Setting Up Your Development Environment
A well-prepared development environment ensures seamless plugin creation. This stage involves gathering tools and installing WordPress locally for testing and code execution.
Tools You Need To Get Started
- Code Editor: Use a reliable editor like Visual Studio Code or Sublime Text. These provide syntax highlighting, debugging, and file organization.
- Local Server Environment: Install software like XAMPP, MAMP, or Local by Flywheel to run WordPress locally.
- WordPress Installation Package: Download the latest WordPress version from the official WordPress.org website.
- PHP and MySQL Support: Ensure PHP v8.0 or higher and MySQL or MariaDB are available in your server environment.
- Browser Developer Tools: Leverage built-in browser tools for inspecting and debugging your plugin’s front-end behavior.
- Version Control System: Use Git for code tracking and collaboration.
- Download Local Server Software: Choose a tool like XAMPP for Windows or MAMP for macOS. Install it following its setup instructions.
- Configure Server Settings: Start the server software and configure it to match WordPress requirements (e.g., PHP version and database setup).
- Create a Database: Access your server’s database tool (e.g., phpMyAdmin), create a new MySQL database, and name it for your WordPress project.
- Set Up WordPress Files: Move the downloaded WordPress files into the specified server directory (e.g.,
htdocs
for XAMPP). - Run WordPress Installation: Open your browser, navigate to
http://localhost/[project-folder]
, and follow the WordPress installation wizard to configure site details and the database.
After completing these steps, your environment is ready for WordPress plugin development.
Building Your First WordPress Plugin
Learning how to build a WordPress plugin begins with understanding the structure, writing the header, and implementing core functionality. Follow these steps to create your first custom plugin and expand your website’s capabilities.
Understanding Basic Plugin Structure
Start by creating a folder in the wp-content/plugins
directory. Use a meaningful name, such as my-first-plugin
, to organize your work. Inside this folder, create a main PHP file with the same name as your plugin folder, e.g., my-first-plugin.php
.
Include any additional files like CSS, JavaScript, or image assets within subdirectories such as css
, js
, or assets
. Maintain this structure to keep your plugin modular and manageable.
The core structure should look like this:
wp-content/
plugins/
my-first-plugin/
my-first-plugin.php
css/
js/
assets/
Writing The Plugin Header
Open the main PHP file and define your plugin’s header information. Headers provide WordPress with metadata about your plugin, including its name, version, author, and description. This data ensures compatibility and makes the plugin identifiable in the dashboard.
Below is a sample header block:
<?php
/*
Plugin Name: My First Plugin
Plugin URI: https://example.com/plugins/my-first-plugin
Description: A simple custom plugin for WordPress.
Version: 1.0
Author: Your Name
Author URI: https://example.com
License: GPL2
*/
?>
Replace the placeholder values with accurate and relevant details for your plugin. Ensure these comment lines are at the top of your PHP file.
Adding Core Functionality
Define the actions or behaviors your plugin introduces to the site. Use WordPress hooks, such as add_action
or add_filter
, to implement specific functionalities without modifying the core files.
For example, add a custom message to the footer using the wp_footer
hook:
function custom_footer_message() {
echo '<p style="text-align: center;">Powered by My First Plugin</p>';
}
add_action('wp_footer', 'custom_footer_message');
Place your functions and hooks within the main plugin file or separate them into modules for large-scale plugins. Following this approach ensures that the plugin remains scalable and easy to maintain.
Enhancing Your Plugin Features
Extending functionality improves your plugin’s usability and customization. Implement advanced features like hooks, filters, shortcodes, and options to provide flexibility and meet diverse user needs.
Using Hooks And Filters
Hooks and filters allow interaction with WordPress’s core and other plugins. With hooks, you attach custom functions at specified points, while filters modify data before display or processing.
- Action Hooks: Execute your code at specific points (e.g., after loading headers). Use the
add_action()
function to link your action. Example:
add_action('wp_footer', 'custom_footer_message');
function custom_footer_message() {
echo '<p>Custom Footer Message</p>';
}
- Filters: Alter content or variables dynamically. Use
add_filter()
to apply changes. Example:
add_filter('the_content', 'custom_content_filter');
function custom_content_filter($content) {
return $content . '<p>Additional content appended.</p>';
}
Focus on debugging interactions to avoid conflicts with other plugins or themes.
Integrating Custom Shortcodes
Shortcodes let users add functionality or formatted content by inserting a simple tag into posts, pages, or widgets. Define shortcodes in your plugin to increase user convenience.
- Creating a Shortcode: Use the
add_shortcode()
function to register a tag. Example:
add_shortcode('custom_button', 'render_custom_button');
function render_custom_button() {
return '<button>Click Me</button>';
}
- Parameter Handling: Allow attributes in shortcodes to offer dynamic functionality. Example:
add_shortcode('dynamic_button', 'render_dynamic_button');
function render_dynamic_button($atts) {
$attributes = shortcode_atts(array('text' => 'Click'), $atts);
return '<button>' . esc_html($attributes['text']) . '</button>';
}
Always sanitize user inputs to improve security.
Managing Plugin Options
Include options in a settings page to allow users to configure your plugin without editing code. Use the WordPress Settings API for standardized option handling.
- Adding a Menu: Use
add_options_page()
to create a menu item under “Settings.” Example:
add_action('admin_menu', 'custom_plugin_menu');
function custom_plugin_menu() {
add_options_page('Plugin Settings', 'My Plugin', 'manage_options', 'my-plugin', 'custom_plugin_settings_page');
}
- Creating a Settings Page: Build the page’s structure with HTML or WordPress functions. Example:
function custom_plugin_settings_page() {
echo '<h1>Plugin Settings</h1>';
echo '<form method="post" action="options.php">';
settings_fields('my_plugin_group');
do_settings_sections('my_plugin');
submit_button();
echo '</form>';
}
- Registering Settings: Use
register_setting()
to save configuration data. Example:
add_action('admin_init', 'register_my_plugin_settings');
function register_my_plugin_settings() {
register_setting('my_plugin_group', 'my_plugin_option');
}
Ensure easy usability by grouping related settings logically. Add default values to prevent errors from uninitialized options.
Testing And Debugging Your Plugin
Thorough testing and debugging are essential to ensure your WordPress plugin functions smoothly across different environments. Focus on compatibility, functionality, and resolving issues for a seamless user experience.
Testing For Compatibility
Verify that your plugin works across various WordPress versions, themes, and plugins. Compatibility testing helps prevent conflicts and ensures broader usability.
- Test Across WordPress Versions: Use a staging site to test your plugin on multiple WordPress versions, especially the latest release and the one before it. This ensures users running older versions won’t encounter issues.
- Check Theme Interactions: Install a variety of popular themes and check whether your plugin adapts consistently, especially if it interacts with layouts or visuals.
- Assess Plugin Conflicts: Activate your plugin alongside others, especially widely-used ones like Yoast SEO or WooCommerce. Identify and resolve any conflicts that arise during simultaneous use.
Set up a controlled environment to simulate real-world scenarios without risking live website data. Tools like WP Sandbox or Local offer isolated spaces for effective testing.
Debugging Common Issues
Address common bugs during plugin development by leveraging debugging tools and structured workflows. Debugging ensures your plugin performs flawlessly under different circumstances.
- Enable WordPress Debugging: Use
WP_DEBUG
in the wp-config.php file to display PHP warnings and errors. Setdefine('WP_DEBUG', true);
to identify issues during development. - Analyze Logs: Examine the
debug.log
file for detailed error information. Check for deprecated functions or unsanitized inputs. - Browser Developer Tools: Inspect JavaScript errors using browser tools like Chrome DevTools. Fix conflicts or script loading issues based on error messages.
- Utilize Debugging Plugins: Install plugins like Query Monitor or Debug Bar to monitor database queries, scripts, and hooks, ensuring efficient code execution.
- Test User Inputs: Validate and sanitize every form input to prevent vulnerabilities. This improves both usability and security.
Approach debugging methodically by prioritizing critical errors and keeping changes logged for future reference. Employ Git Branching to revert code if needed during issue resolution.
Publishing Your WordPress Plugin
Publishing your WordPress plugin ensures it reaches a wider audience and benefits other users. Following the proper steps helps maintain quality and visibility within the WordPress ecosystem.
Preparing Your Plugin For Release
Prepare your plugin by refining its structure, compatibility, and documentation. These elements ensure it meets WordPress standards and user expectations.
- Optimize Code Structure: Include only necessary files and keep your code modular. Remove unused assets, debugging functions, and unnecessary comments to enhance performance.
- Check Compatibility: Test across various PHP versions and WordPress releases, ensuring your plugin works with major themes and popular plugins.
- Add Plugin Documentation: Write a detailed readme.txt file. Include the plugin’s name, description, installation steps, FAQs, and changelog. Use the WordPress Readme Validator to check formatting.
- Localize The Plugin: Use WordPress functions like
__()
and_e()
to make your plugin translation-ready. Generate a .pot file for translation using tools such as Poedit or WP CLI’s i18n feature. - Verify Security: Sanitize user inputs with
sanitize_*()
functions, escape outputs usingesc_*()
functions, and use nonces for form validation.
Submitting To The WordPress Plugin Directory
Submitting your plugin to the WordPress plugin directory increases its discoverability to millions of users.
- Create a WordPress.org Account: Register on WordPress.org if you don’t already have an account.
- Review Plugin Guidelines: Read the plugin directory guidelines. Ensure your plugin strictly adheres to these rules, especially regarding security and licensing.
- Submit Your Plugin: Go to the Add Your Plugin page and fill out the submission form. Provide the plugin’s name, description, and ZIP file containing plugin files.
- Await Approval: The WordPress team reviews your submission for guideline compliance. The review process typically takes 2-7 days.
- Set Up SVN Repository: Once approved, upload your plugin files to the assigned SVN repository using tools like TortoiseSVN or Git-SVN bridge. Structure the tags, trunk, and branches directories correctly for versioning.
- Publish Release Notes: Update your plugin’s directory page with the latest changelog and release details. Monitor feedback and provide prompt updates as needed.
Focus on clarity, organization, and user-centered design to maximize your plugin’s impact and ensure its long-term success.
Conclusion
Creating a WordPress plugin empowers you to customize your website in ways that align perfectly with your goals. By following a structured approach and leveraging the tools and best practices outlined, you can build a functional, secure, and scalable plugin that enhances your site’s performance. Whether you’re adding unique features or solving specific challenges, your custom plugin can make a lasting impact. Take the first step and turn your ideas into reality—your WordPress site will thank you.
Frequently Asked Questions
What is a WordPress plugin?
A WordPress plugin is an add-on that enhances the functionality of a WordPress website. It allows you to add features or integrate third-party services without altering the core WordPress code, making customization easy and efficient.
Why should I create my own WordPress plugin?
Creating your own plugin provides tailored features, improved security, better performance, and a competitive edge. It also reduces compatibility issues and allows you to build solutions that align with your specific website goals.
What tools do I need to create a WordPress plugin?
You’ll need a code editor (e.g., Visual Studio Code), a local server environment (e.g., XAMPP or MAMP), a WordPress installation, PHP and MySQL support, browser developer tools, and optionally, a version control system like Git.
Where do I create my WordPress plugin files?
WordPress plugins are created in the wp-content/plugins
directory. You can create a folder for your plugin and include a main PHP file along with subdirectories for CSS, JavaScript, and other assets.
What is a plugin header in WordPress?
The plugin header is metadata in the main plugin PHP file. It includes details such as the plugin’s name, version, author, and description. This information is displayed in the WordPress dashboard.
How do action hooks and filters enhance plugins?
Action hooks execute specific code at predefined points, while filters allow you to modify content dynamically. Both enable you to extend and customize plugin functionality without altering the core WordPress code.
How can I debug my WordPress plugin?
You can debug your plugin by enabling WordPress debugging, analyzing error logs, using browser developer tools, and testing with debugging plugins. Validate user inputs and test in various environments to ensure compatibility.
How do I test my WordPress plugin for compatibility?
Test your plugin with different WordPress versions, themes, and plugins to identify any conflicts. Ensure it works smoothly across various environments and use feedback tools to fix any issues that arise.
How do I publish my WordPress plugin?
To publish your plugin, optimize its structure, localize it, add documentation, and ensure compatibility. Create a WordPress.org account, submit your plugin to the directory, and follow the SVN repository setup instructions.
Are WordPress plugins secure?
Plugins can be secure if you follow best practices like sanitizing inputs, validating data, and minimizing vulnerabilities. Regularly update plugins and use debugging tools to keep them secure.