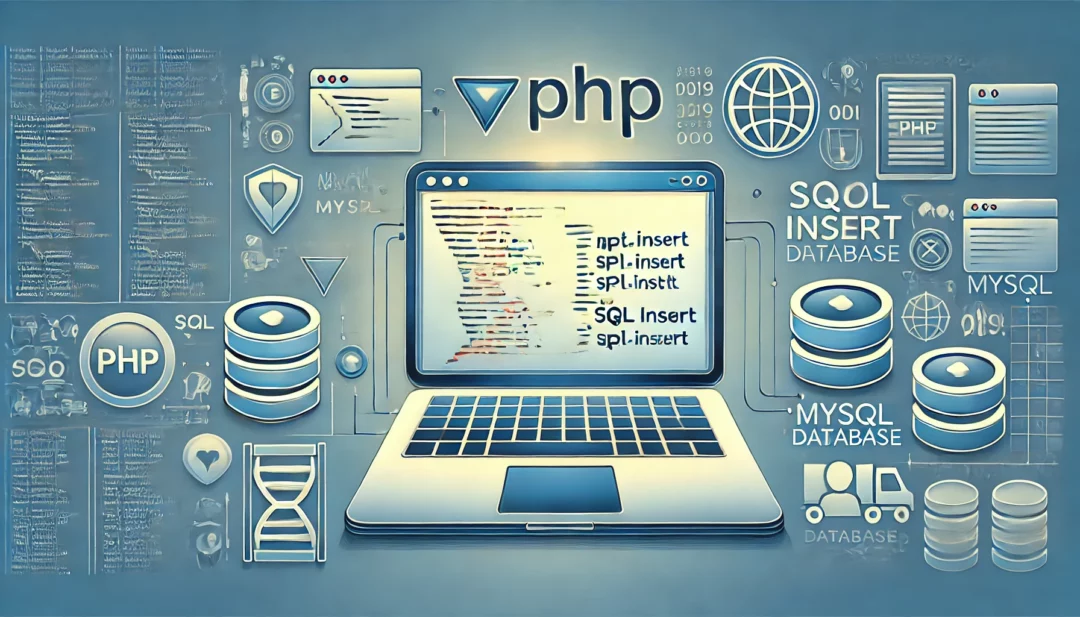
Inserting data into a MySQL database using PHP is a fundamental skill for building dynamic web applications. Whether you’re creating a user registration form or managing a product catalog, this process is essential for storing and managing information efficiently. By combining PHP’s server-side capabilities with MySQL’s robust database management, you can create seamless data interactions.
To get started, you’ll need to follow a few straightforward steps. First, establish a connection to your MySQL database using PHP. Next, prepare an SQL INSERT query to define the data you want to add. Finally, execute the query and confirm the data has been successfully inserted. With these steps, you’ll be able to handle database operations quickly and effectively.
Understanding PHP and MySQL
PHP and MySQL work together to build dynamic and interactive web applications. PHP handles server-side logic, while MySQL manages and stores structured data. Combining these technologies allows you to create, retrieve, update, and delete data in a database effectively.
MySQL operates as the database management system (DBMS), organizing data into tables with rows and columns. PHP communicates with MySQL using functions such as mysqli
or PDO
to execute SQL queries. For example, storing user details in a registration form involves PHP capturing input and MySQL saving it into a database table.
Security is an essential consideration when managing database operations. Using prepared statements
in PHP helps prevent SQL injection, ensuring safe and reliable communication between PHP and MySQL.
Setting Up the Environment
Prepare a functional development environment to insert data into a MySQL database using PHP. Ensure all necessary components are installed and configured properly.
Installing PHP
Download the latest PHP version from the official PHP website. Select the version compatible with your operating system.
- Install PHP on Windows: Use tools like XAMPP or WampServer, as they include PHP preconfigured with other required services.
- Install PHP on macOS: Install Homebrew, then use the
brew install php
command in your terminal. - Install PHP on Linux: Use terminal commands specific to your distribution, such as
sudo apt-get install php
for Ubuntu.
Verify that PHP is installed successfully using the php -v
command in your terminal or command prompt.
Installing MySQL
Download MySQL Community Server from the official MySQL website. Follow the installation steps for your operating system.
- Install MySQL on Windows: Use the MySQL Installer to guide you through the installation process, configuring the root password and default server settings.
- Install MySQL on macOS: Use Homebrew with the
brew install mysql
command. - Install MySQL on Linux: Enter
sudo apt-get install mysql-server
for Debian-based distributions.
Log in to MySQL using the mysql -u root -p
command to confirm successful installation.
Configuring a Local Server
Set up a local server to test and execute PHP scripts with database connectivity.
- Using XAMPP: Download and install XAMPP, which bundles Apache, PHP, and MySQL. Start the Apache and MySQL modules in the XAMPP control panel.
- Using WAMP: For Windows, install WampServer to easily manage services like Apache and MySQL together.
- Using MAMP: On macOS, use MAMP for a straightforward local environment setup.
Place your PHP files in the designated folder (e.g., htdocs
in XAMPP). Access files via http://localhost/your-file.php
in your web browser for testing.
Creating a MySQL Database
A MySQL database serves as the central repository for storing and managing structured data used in web applications. For inserting data through PHP, outline the right structure and logic for your database to ensure efficient storage and retrieval.
Designing Tables for Data Storage
- Understand Your Data Requirements
Determine the type of data your application handles, such as user information or product details. Based on this, identify the columns and their specific data types like VARCHAR
for text, INT
for numbers, and DATE
for dates. For example, a “users” table may include id (INT)
, name (VARCHAR)
, and email (VARCHAR)
.
- Define Primary Keys and Constraints
Assign a PRIMARY KEY
to uniquely identify records, such as an auto-incrementing id
column, for efficient management. Use constraints like NOT NULL
for mandatory fields and UNIQUE
for attributes like email
that shouldn’t repeat.
- Create a Table Using SQL
Use the SQL CREATE TABLE
statement to design your table:
CREATE TABLE users (
id INT AUTO_INCREMENT PRIMARY KEY,
name VARCHAR(100) NOT NULL,
email VARCHAR(100) UNIQUE NOT NULL,
created_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP
);
This table stores user data with constraints and a timestamp column for record creation dates.
- Normalize the Database
Avoid redundant data by following normalization rules. For example, separate product categories into another table instead of repeating them within a single table.
- Use the
INSERT INTO
Statement
Prepare a simple INSERT INTO
query to add data to your table. For example:
INSERT INTO users (name, email)
VALUES ('John Doe', '[email protected]');
This query inserts a new user into the “users” table.
- Include Multiple Records
Insert multiple records simultaneously using a single query:
INSERT INTO users (name, email)
VALUES
('Jane Smith', '[email protected]'),
('Adam White', '[email protected]');
This method reduces database round trips for bulk data handling.
- Utilize Prepared Statements for Security
Protect your database against SQL injection by using prepared statements in your PHP code. For instance:
$stmt = $conn->prepare("INSERT INTO users (name, email) VALUES (?, ?)");
$stmt->bind_param("ss", $name, $email);
$stmt->execute();
This approach ensures that the data is properly sanitized before reaching the MySQL server.
- Handle Errors Effectively
Monitor errors that might occur during insertion. Use PHP’s mysqli error
property or write custom error messages in your script to troubleshoot failed queries:
if (!$stmt->execute()) {
echo "Error: " . $stmt->error;
}
By following these steps, build a robust foundation for managing database interactions using PHP and MySQL, stressing both efficiency and security.
Writing PHP Code to Insert Data
Writing PHP code to insert data into a MySQL database is a critical process for building dynamic web applications. By following structured steps, you can efficiently and securely execute data insertion tasks.
Connecting PHP to MySQL
Connect PHP to MySQL using either the MySQLi extension or PDO (PHP Data Objects). Both methods establish a secure communication channel between PHP and your database. Use MySQLi for procedural or object-oriented approaches, and choose PDO for better flexibility across different databases.
Example: Using MySQLi (Object-Oriented):
$servername = "localhost";
$username = "root";
$password = "your_password";
$database = "your_database";
$conn = new mysqli($servername, $username, $password, $database);
if ($conn->connect_error) {
die("Connection failed: " . $conn->connect_error);
}
echo "Connected successfully";
Replace "your_password"
and "your_database"
with those matching your setup. For PDO, adjust syntax accordingly:
try {
$conn = new PDO("mysql:host=localhost;dbname=your_database", $username, $password);
$conn->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION);
echo "Connected successfully";
} catch (PDOException $e) {
echo "Connection failed: " . $e->getMessage();
}
For security, store sensitive credentials in a configuration file outside the webroot.
Writing the Data Insertion Script
Create an SQL INSERT query in the PHP script to include data into your MySQL database. Use a prepared statement to prevent SQL injection and improve query execution security.
Example: Data Insertion Script with MySQLi:
$sql = $conn->prepare("INSERT INTO users (name, email) VALUES (?, ?)");
$sql->bind_param("ss", $name, $email);
$name = "John Doe";
$email = "[email protected]";
if ($sql->execute()) {
echo "Data inserted successfully";
} else {
echo "Error: " . $sql->error;
}
$conn->close();
Example: Using PDO:
$stmt = $conn->prepare("INSERT INTO users (name, email) VALUES (:name, :email)");
$stmt->bindParam(':name', $name);
$stmt->bindParam(':email', $email);
$name = "Jane Doe";
$email = "[email protected]";
if ($stmt->execute()) {
echo "Data inserted successfully";
} else {
echo "Error occurred";
}
$conn = null;
Always replace placeholders like "users"
, "name"
, and "email"
with your database table and column names.
Handling Form Data for Dynamic Inputs
Retrieve user inputs dynamically from an HTML form using PHP’s $_POST
superglobal. Sanitize and validate all inputs to enhance security and functionality.
Example: Collecting and Inserting Form Data:
HTML Form:
<form method="post" action="insert.php">
<label for="name">Name:</label>
<input type="text" id="name" name="name" required>
<label for="email">Email:</label>
<input type="email" id="email" name="email" required>
<button type="submit">Submit</button>
</form>
PHP Processing Script (insert.php
):
$name = htmlspecialchars($_POST['name']);
$email = htmlspecialchars($_POST['email']);
$sql = $conn->prepare("INSERT INTO users (name, email) VALUES (?, ?)");
$sql->bind_param("ss", $name, $email);
if ($sql->execute()) {
echo "Form data inserted successfully";
} else {
echo "Error: " . $sql->error;
}
Validate data (e.g., validate email structure) and handle errors to avoid incorrect or malicious inputs.
Debugging and Testing the Code
Debugging and testing ensure your PHP script correctly inserts data into the MySQL database. Identifying errors and validating functionality minimizes issues during deployment. Below are steps to effectively debug and test the process.
Common Errors and Their Solutions
Addressing common errors enhances reliability. Here are typical issues and how to resolve them:
- Database Connection Failure
Verify credentials such as hostname, username, password, and database name. Test the connection using mysqli_connect_error()
or a PDO exception to pinpoint errors.
Example:
if (!$conn) {
die("Connection failed: " . mysqli_connect_error());
}
- SQL Syntax Errors
Fix these by reviewing your query and ensuring it adheres to SQL standards. Use a tool like phpMyAdmin to test the query directly, identifying any syntax issues.
- Undefined Variables or Parameters
Check for typos in variable names or uninitialized parameters. Enable error_reporting(E_ALL)
to display notice-level errors.
- Duplicate Entries
Address primary key violations by defining unique constraints carefully. Use INSERT IGNORE
or ON DUPLICATE KEY UPDATE
for conditional data insertion.
- SQL Injection Risks
Enhance security by implementing prepared statements. Avoid embedding user input directly into SQL queries.
Example with Prepared Statement:
$stmt = $conn->prepare("INSERT INTO users (name, email) VALUES (?, ?)");
Testing the Data Insertion Process
Systematic testing confirms accurate data handling. Follow these steps to test the insertion process:
- Add Test Data
Create an HTML form to simulate user inputs. Include all required fields matching your database schema.
Example Form:
<form method="POST" action="insert.php">
<input type="text" name="name" placeholder="Enter name">
<input type="email" name="email" placeholder="Enter email">
<button type="submit">Submit</button>
</form>
- Examine Backend Logs
Enable error logging in PHP to track issues. Use ini_set('log_errors', 1)
and define a log file for error storage.
- Verify Database Records
Query the database manually to confirm entries. Use SELECT * FROM table_name
to check for proper insertion and data integrity.
- Perform Edge Case Tests
Input unexpected data like special characters, empty fields, or script tags to test validation and sanitization mechanisms.
Example Validation:
if (!filter_var($email, FILTER_VALIDATE_EMAIL)) {
echo "Invalid email format";
}
- Automate Testing
Use tools like PHPUnit to script repetitive tests. This ensures consistency and saves time for larger projects.
Enhancing Security
Ensuring the security of your database operations is critical when inserting data into a MySQL database using PHP. Implement secure coding practices to protect user data and prevent vulnerabilities like SQL injection.
Using Prepared Statements
Prepared statements help prevent SQL injection by separating SQL logic from user input. Instead of embedding input directly into your query, use placeholders to bind and execute parameters safely.
- Prepare the SQL Query: Write an SQL query with placeholders for dynamic values. For example:
$sql = "INSERT INTO users (username, email) VALUES (?, ?)";
- Bind Parameters: Use a method like
bind_param()
(MySQLi) orbindValue()
(PDO) to bind user input securely to the placeholders:
MySQLi Example:
$stmt = $conn->prepare($sql);
$stmt->bind_param("ss", $username, $email);
PDO Example:
$stmt = $conn->prepare($sql);
$stmt->bindValue(1, $username, PDO::PARAM_STR);
$stmt->bindValue(2, $email, PDO::PARAM_STR);
- Execute the Statement: Run the prepared statement to insert data. For MySQLi, use the
execute()
method, while for PDO, useexecute()
orquery()
. Prepared statements handle escaping and encoding, which reduces the risk of malicious input altering your query logic.
Validating Input Data
Input data validation ensures only appropriate and safe data is processed. Without this step, errors or malicious data could lead to injection, corruption, or application crashes.
- Sanitize Data: Remove harmful characters using PHP functions like
filter_var()
orhtmlspecialchars()
. For example, sanitize email inputs as below:
$email = filter_var($_POST['email'], FILTER_SANITIZE_EMAIL);
- Validate Data Format: Ensure inputs match expected formats using PHP validation filters or regex. For example:
- Validate email:
if (!filter_var($email, FILTER_VALIDATE_EMAIL)) {
echo "Invalid email format.";
}
- Validate numeric input:
if (!is_numeric($age)) {
echo "Age must be numeric.";
}
- Check Boundaries: Restrict inputs within practical or valid ranges, such as limiting string lengths or age values. For instance, validate username length:
if (strlen($username) < 3
|
|
strlen($username) > 20) {
echo "Username must be between 3 and 20 characters.";
}
- Handle Mandatory Fields: Verify all required fields are filled before processing. Check each field individually or use a loop through form inputs.
Integrating these practices alongside prepared statements reinforces your application’s security and ensures accurate, reliable database interactions.
Conclusion
Mastering how to insert data into a MySQL database using PHP equips you with the skills to build dynamic, secure, and efficient web applications. By combining PHP’s server-side capabilities with MySQL’s robust data management, you can handle user inputs, store critical information, and ensure seamless database interactions.
With a focus on security and proper coding practices, you can safeguard your applications from vulnerabilities while maintaining data integrity. Whether you’re developing a simple form or a complex system, understanding this process is a valuable step toward creating reliable and scalable solutions.
Frequently Asked Questions
What is the purpose of using PHP with MySQL?
PHP and MySQL work together to create dynamic web applications. PHP handles server-side logic, while MySQL manages and stores structured data. This combination is ideal for building features like user registration systems and product catalogs.
How do I establish a connection between PHP and MySQL?
You can connect PHP to MySQL using extensions like MySQLi or PDO. Both offer functions to establish a secure connection by providing database credentials and server configurations.
What are prepared statements, and why are they important?
Prepared statements separate SQL queries from user input, making them a security measure against SQL injection attacks. They also improve the efficiency of executing repeated queries.
Can I use XAMPP, WAMP, or MAMP to test PHP scripts?
Yes, these local server tools allow you to set up a development environment for testing PHP scripts with MySQL database connectivity on your computer.
How do I insert data into a MySQL database using PHP?
Use an SQL INSERT INTO
statement in your PHP script. Establish a database connection, prepare the query, bind parameters (if using prepared statements), and execute it to insert data securely.
What are some common errors when inserting data into MySQL with PHP?
Typical errors include invalid database credentials, SQL syntax issues, connection failures, or duplicate entries. Debugging involves checking logs, reviewing code syntax, and testing edge cases.
How do I validate and sanitize input from forms in PHP?
Use PHP functions like filter_var()
to validate inputs (e.g., email format). Sanitization removes harmful characters to prevent malicious inputs, ensuring secure data interactions.
How do I prevent SQL injection when using PHP and MySQL?
Prevent SQL injection by always using prepared statements or parameterized queries. Avoid directly embedding user inputs into SQL queries.
What is PDO, and how is it different from MySQLi?
PDO (PHP Data Objects) is a database access library supporting multiple databases, not just MySQL. MySQLi is specific to MySQL but offers better performance for MySQL-only projects. Both can be used for secure queries.
How can I debug PHP and MySQL scripts?
Debugging involves enabling error reporting in PHP, reviewing backend logs, checking database records, testing inputs, and fixing issues like missing fields or invalid queries systematically.
Why is database structure important?
A well-structured database ensures efficient data storage and retrieval. Proper indexing, normalization, and primary key definitions help reduce redundancy and improve query performance.
What is normalization in database design?
Normalization organizes database tables to avoid redundant data and maintain consistency. It ensures there are no duplicate fields and that relationships between data are properly structured.
How do I secure sensitive database credentials in PHP?
Store database credentials in a separate configuration file outside the web root directory. Use environment variables or encryption methods to keep them secure.
Can I insert multiple rows into a MySQL database simultaneously?
Yes, you can insert multiple rows using a single INSERT INTO
query by separating values with commas. This improves efficiency compared to inserting rows one by one.
What are the benefits of testing a PHP-MySQL integration?
Testing ensures accurate data insertion, identifies errors, and verifies functionality. It also helps address edge cases and fosters reliable application behavior, especially in large projects.