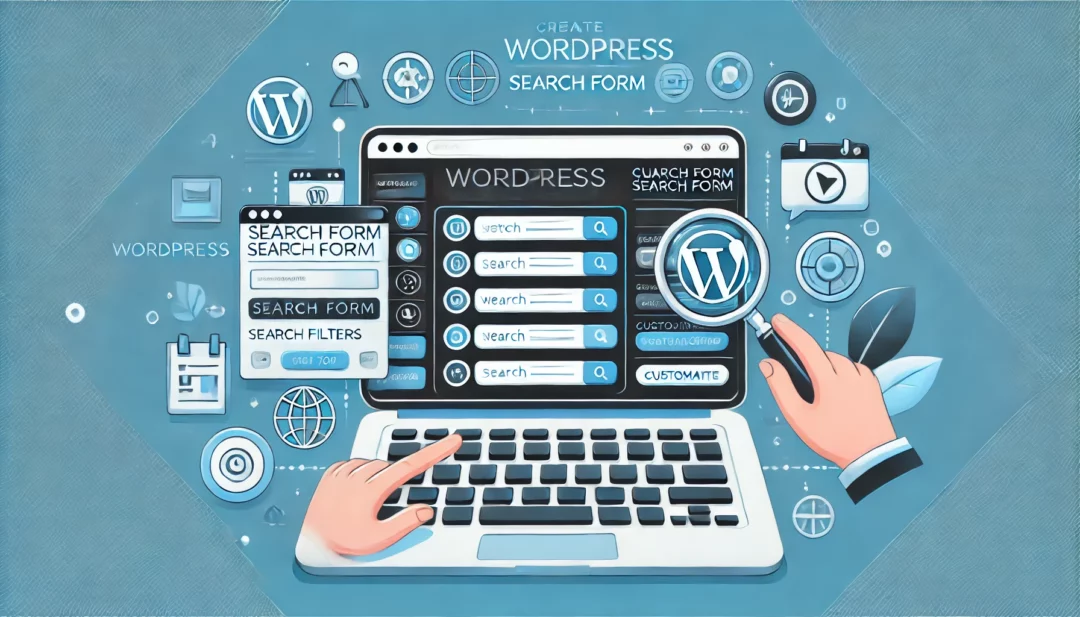
Creating a custom WordPress search form might sound complex, but it’s easier than you think with the right steps. Whether you’re enhancing user experience or tailoring functionality to fit your site’s unique needs, a personalized search form can make a big difference. By customizing it, you ensure your visitors find exactly what they’re looking for, boosting engagement and satisfaction.
In this guide, you’ll learn how to build a search form that aligns perfectly with your website’s design and purpose. From setting up the basic structure to adding advanced features, every step is broken down so you can follow along effortlessly. With just a bit of coding and creativity, you’ll have a fully functional search form that stands out and works seamlessly.
Why Customize A WordPress Search Form?
Customizing a WordPress search form enhances both functionality and aesthetics. The default search form may not fully address your website’s unique needs or user behavior. Tailoring it ensures better compatibility with your site’s design and usability.
- Improve Search Accuracy: A customized search form can filter irrelevant results. For example, you might include or exclude specific post types, categories, or tags to refine searches.
- Enhance User Experience: A form tailored to your users’ needs makes navigation smoother. Custom placeholders, labels, and layout adjustments create an intuitive interface.
- Match Site Design: Ensuring the search form aligns with your site’s visual elements improves professionalism. You can modify colors, fonts, or button styles to fit your theme.
- Add Functionality: Expand the form’s capabilities by integrating features like AJAX suggestions, search by filters, or autocomplete options. These adjustments boost engagement.
- Address SEO Goals: A targeted search form reduces bounce rates by guiding visitors to relevant content. Improved discovery aligns user searches with available resources.
A tailored search form seamlessly combines practicality and design, adapting to your site’s goals and audience requirements.
Tools And Prerequisites
Creating a custom WordPress search form requires specific tools and a foundational skillset. Ensuring you’re equipped with the correct resources simplifies the process and minimizes potential errors.
WordPress Installation
Have a fully functional WordPress site set up. This includes installing WordPress on a web server and configuring it with a unique domain. Use hosting providers like Bluehost or SiteGround, which offer straightforward installation. Confirm your WordPress version is up-to-date for compatibility and security.
Text Editor And FTP Access
Use a reliable text editor, such as Sublime Text, Atom, or VS Code, to modify code efficiently. These editors offer syntax highlighting and error detection. Ensure you have access to an FTP client like FileZilla or Cyberduck for uploading changes directly to your WordPress files. Verify your hosting provider supplies FTP credentials, including server address, username, and password.
Basic Knowledge Of HTML And PHP
Understand the fundamentals of HTML for structuring your search form and PHP for embedding search functionalities into WordPress. Identify PHP functions like get_search_form()
and how they interact with templates. If you’re unfamiliar, refer to beginner tutorials on HTML and PHP basics to build confidence before proceeding.
Step 1: Create A Child Theme
Creating a child theme ensures your custom WordPress search form changes don’t overwrite essential website files during updates. This dedicated framework separates your modifications from core theme files, maintaining site functionality and simplifying future updates.
Why Use A Child Theme?
A child theme allows you to safely modify or extend an existing WordPress theme. Direct changes to the parent theme can be lost during updates, but a child theme preserves your customizations. It also provides a structured way to test updates or new features without affecting your main site.
- Create A New Folder
Access your WordPress directory using FTP or a file manager. Navigate to /wp-content/themes/
and create a new folder. Name it descriptively, such as parent-theme-child
, where “parent-theme” matches your active parent theme.
- Add A
style.css
File
Inside the new folder, create a file named style.css
. Include the following template header, replacing placeholders with your specific details:
/*
Theme Name: Parent Theme Child
Template: parent-theme
*/
The Template
value must exactly match your parent theme’s directory name.
- Add A
functions.php
File
To ensure your child theme inherits styles and functions from the parent, create a functions.php
file in your child theme folder. Add the following code:
<?php
add_action( 'wp_enqueue_scripts', 'enqueue_parent_styles' );
function enqueue_parent_styles() {
wp_enqueue_style( 'parent-style', get_template_directory_uri() . '/style.css' );
}
?>
- Activate Your Child Theme
Log in to your WordPress dashboard. Under “Appearance” > “Themes,” locate your child theme and click “Activate.” Confirm by visiting your site to check that it loads properly with no styling issues.
These basic steps create a foundation for customizing your WordPress search form and other theme features.
Step 2: Add Custom Search Functionality
Adding custom search functionality allows you to enhance how users interact with your WordPress site’s search feature. This step involves editing WordPress template files and writing PHP code to integrate tailored search behaviors.
Editing The searchform.php File
Locate the searchform.php
file in your theme folder. If the file doesn’t exist, create one within your child theme directory.
- Add Basic HTML Structure: Include HTML elements like a
<form>
tag with themethod
attribute set toget
. For example:
<form role="search" method="get" id="searchform" action="<?php echo home_url( '/' ); ?>">
<input type="text" name="s" id="s" placeholder="Search...">
<button type="submit" id="searchsubmit">Search</button>
</form>
Ensure the action
attribute refers to your site’s home URL using home_url()
.
- Style the Form: Apply basic CSS rules to align the design with your theme’s aesthetics. Edit the
style.css
file in your child theme for styling.
Writing Custom PHP Code
Modify PHP code to control search functionality. Open the functions.php
file in your child theme directory.
- Pre-Filter Search Queries: Use the
pre_get_posts
hook to refine search results. Add this code:
function filter_search_results( $query ) {
if ( $query->is_search && !is_admin() ) {
$query->set( 'post_type', array( 'post', 'page' ) ); // Limit results to posts and pages
$query->set( 'posts_per_page', 10 ); // Define number of results
}
}
add_action( 'pre_get_posts', 'filter_search_results' );
- Exclude Categories from Search: Exclude specific categories by adding:
function exclude_category_from_search( $query ) {
if ( $query->is_search && !is_admin() ) {
$query->set( 'cat', '-5' ); // Replace '5' with the ID of the category to exclude
}
}
add_action( 'pre_get_posts', 'exclude_category_from_search' );
- Enable Custom Fields Search (Optional): Customize search to include metadata fields with:
function search_custom_fields( $query ) {
if ( $query->is_search && !is_admin() ) {
$meta_query = array(
'relation' => 'OR',
array(
'key' => 'custom_field_name',
'value' => $query->query_vars['s'],
'compare' => 'LIKE'
)
);
$query->set( 'meta_query', $meta_query );
}
}
add_action( 'pre_get_posts', 'search_custom_fields' );
Always test your changes to ensure they operate as expected before deploying them live.
Step 3: Style The Search Form
Styling ensures your custom WordPress search form aligns with your site’s aesthetic and improves the user experience. Use custom CSS to add visual consistency and functionality to the search form.
Adding Custom CSS
Define a clear design for your search form using custom CSS. Access the style.css file within your child theme directory using a text editor like Sublime Text or VS Code. Add CSS rules that target the search form’s input fields, submit buttons, and container elements.
- Target Form Elements: Use selectors like
form
,input[type="search"]
, andbutton
to apply styling. For example, adjust field dimensions using properties likewidth
,padding
, andborder
. - Modify Typography: Style text inside the input fields and buttons by defining
font-family
,font-size
, andcolor
. Example:color: #333; font-size: 16px;
. - Apply Hover and Focus States: Enhance interactivity using pseudo-classes like
:hover
and:focus
. Define border changes or background effects for a dynamic experience when users interact with the form. - Ensure Responsiveness: Use media queries (
@media
) to adapt CSS rules for smaller screens. For instance, reduce width or adjust padding on mobile devices.
Use specific class names or IDs to avoid unwanted changes to other site elements. Add comments in the CSS file for better organization and future edits.
Testing And Refining The Design
Test the styled form across multiple devices and browsers to verify proper rendering. Use browser developer tools (F12 key on most browsers) to inspect and tweak styles in real time.
- Verify Alignment: Check if the form aligns with your site’s layout. Adjust properties like
margin
andposition
if necessary. - Fine-Tune Colors: Test visibility of elements like text and buttons against varying backgrounds to ensure accessibility.
- Troubleshoot Issues: Fix overlapping elements or unexpected behaviors caused by conflicting CSS rules. For example, inspect the cascade order to ensure the correct styles are applied.
- Test Functionality: Verify the form functions as expected after styling changes. Ensure search submission and results display correctly.
Iteratively refine your design based on test results for a polished and consistent appearance.
Step 4: Implement And Test
Integrating and validating the custom search form ensures it functions as intended while blending seamlessly with your WordPress site. This step involves embedding the form, verifying its functionality, and addressing any issues.
Adding The Search Form To Your Site
Embed the custom search form into your WordPress site by placing it in the desired template file. Identify the location where you want the form to appear, such as the header, sidebar, or footer. Access the relevant template file in your theme’s directory (e.g., header.php
, sidebar.php
, or footer.php
) using your code editor or the Appearance > Theme Editor in the WordPress admin panel.
Insert the PHP code snippet for the search form into the appropriate section of the template. Use the function get_search_form();
to load the custom searchform.php
file from your child theme. For example:
<?php get_search_form(); ?>
Save the changes, and check your site to confirm the form appears in the correct location.
Testing And Debugging
Test the custom search form to ensure it delivers accurate results and performs well across various scenarios. Perform the following checks:
- Functionality
Enter specific search queries to confirm the form retrieves relevant posts, pages, or custom content types based on your PHP settings. If no results are found or irrelevant results appear, review the modifications in functions.php
.
- Responsiveness
Assess the form’s layout on different devices and screen sizes. Confirm that elements resize properly and the design adapts for mobile, tablet, and desktop views. Adjust CSS if the form isn’t fully responsive.
- Cross-Browser Compatibility
View the form in multiple browsers, including Chrome, Firefox, Safari, and Edge, to identify any design or functionality inconsistencies. Modify the CSS or HTML structure to resolve compatibility issues.
- Error Handling
Test scenarios where the search query returns zero results, displays special characters, or contains too many words. Ensure that error messages and results pages inform the user clearly and align with your site’s design.
- Performance
Check if the search form and query results load quickly, especially when advanced features like AJAX are used. Optimize your search queries if performance issues arise.
Use your WordPress site’s debugging tools or enable WP_DEBUG in the wp-config.php
file to track errors. Correct any issues promptly to ensure a smooth user experience.
Conclusion
Creating a custom WordPress search form allows you to tailor functionality and design to your site’s unique needs. By following a structured approach, you can enhance user experience, improve search accuracy, and align the form with your website’s aesthetic. The process may require some technical know-how, but with the right tools and careful testing, you can achieve a seamless and professional result.
A well-designed search form not only boosts engagement but also helps visitors find what they need quickly. Take the time to refine your customizations, and you’ll create a search experience that truly adds value to your site.
Frequently Asked Questions
What is a custom WordPress search form, and why is it important?
A custom WordPress search form is a tailored search feature designed to meet the specific needs of a website. It enhances functionality, aesthetics, and user experience by improving search accuracy, aligning with a site’s design, and meeting visitor expectations. It can also boost engagement and reduce bounce rates by delivering relevant content quickly.
Do I need programming knowledge to create a WordPress custom search form?
Yes, a basic understanding of HTML, PHP, and CSS is helpful. These skills are necessary to structure the search form, define functionalities, and style its appearance. If you’re unfamiliar, beginner tutorials on these languages can be a good starting point.
Why should I use a child theme when customizing a WordPress search form?
A child theme ensures that your customizations, such as search form changes, won’t be overwritten when the parent theme is updated. This protects your work and allows you to make safe modifications without altering the core theme files.
What tools do I need to create a custom WordPress search form?
You’ll need a fully functional WordPress site, a text editor (like VS Code or Sublime Text), an FTP client for uploading changes, and access to the WordPress directory. Proper hosting, such as Bluehost or SiteGround, is also recommended for an optimal setup.
How do I refine search results on a WordPress site?
You can refine search results by editing the functions.php file in your child theme. This allows you to target specific post types, exclude certain categories, or enable searches for custom fields, ensuring more relevant results for users.
How can I style the custom search form to match my website design?
To style the search form, use custom CSS rules in the style.css file of your child theme. Modify form elements like input fields and buttons, adjust typography, and ensure responsiveness. Test extensively across devices and browsers to maintain a consistent appearance.
What should I test after implementing a custom search form?
After implementation, test the search form’s functionality, responsiveness, cross-browser compatibility, and error handling. Check for performance issues and troubleshoot any problems to ensure a smooth, user-friendly search experience.
Can I add advanced features like AJAX to the search form?
Yes, you can add advanced features such as AJAX-based search suggestions or filters. These improve user experience by delivering real-time results and refined searches, making the search form more dynamic and engaging.
Will customizing the search form improve SEO?
Yes, a well-designed and functional search form can improve SEO by reducing bounce rates and guiding users to relevant content. This enhances visitor engagement, which can positively impact your site’s search engine rankings.
How long does it take to create and test a custom WordPress search form?
The time required depends on your familiarity with WordPress, coding, and customization tools. Beginners might take longer, but with step-by-step guidance, most users can complete and test a basic setup within a few hours or a day.