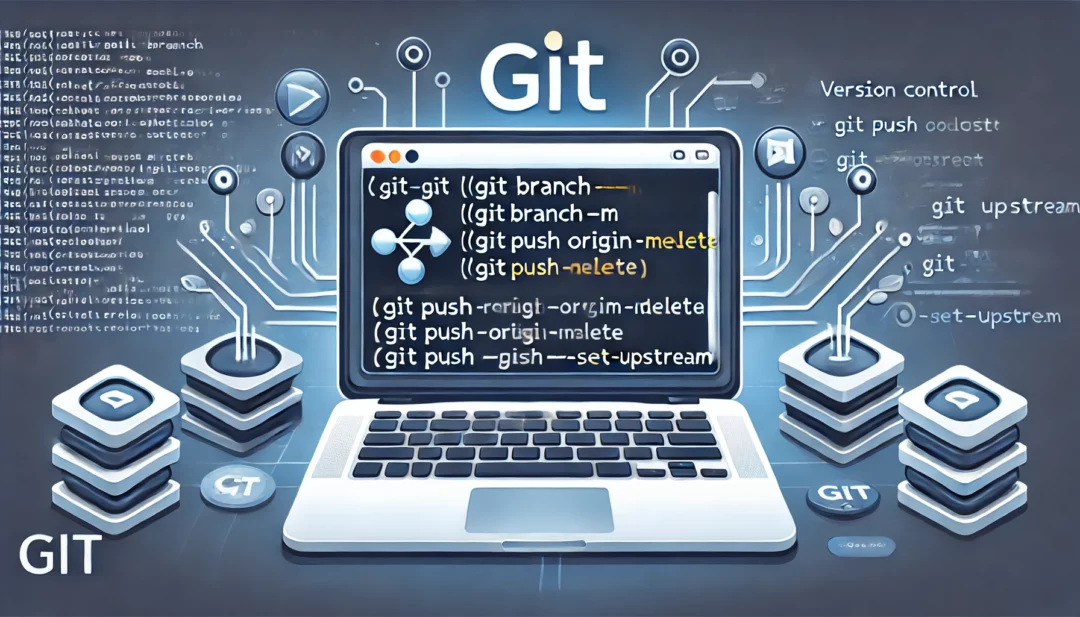
Renaming a Git branch might seem tricky at first, but it’s a straightforward process once you know the steps. Whether you’re cleaning up branch names for clarity or fixing a typo, Git offers simple commands to get the job done. By learning how to rename a branch, you’ll keep your repository organized and easier to manage.
You’ll start by switching off the branch you want to rename, ensuring it’s not currently checked out. Then, use the git branch -m
command to rename it locally. Finally, if the branch is pushed to a remote repository, you’ll delete the old remote branch and push the renamed one. These steps ensure a smooth transition without breaking your workflow.
With just a few commands, you can rename branches like a pro, keeping your Git projects clean and professional. Let’s dive into the details to make this process even easier.
Understanding Git Branches
Git branches serve as independent environments that let you experiment, develop features, or fix bugs without affecting the main project. They allow parallel workflows by enabling you to separate your code changes. Managing branches effectively leads to a more organized and collaborative development process.
Purpose Of Branches
Branches help streamline version control. They allow you to isolate specific work, such as new features or debugging, from the main codebase. For example, you can create a branch for a feature like “user-authentication” while keeping the main branch stable.
Types Of Branches
Branches in Git can be categorized based on purpose:
- Main Branch: Usually named
main
ormaster
, it holds the production-ready code. - Feature Branches: Used for developing specific features, commonly named descriptively (e.g.,
feature/login-system
). - Bug Fix Branches: Focused on debugging and typically named with related identifiers (e.g.,
fix/api-timeout-error
). - Release Branches: Used for preparing code for deployment, often named based on the version release (e.g.,
release/v1.2
).
Branch Workflows
Common workflows associated with Git branches include:
- Creating Branches: You can use
git branch <branch_name>
to create a new branch. For example, typinggit branch feature-auth
creates a feature branch namedfeature-auth
. - Switching Branches: Run
git checkout <branch_name>
orgit switch <branch_name>
to move between branches, such as switching frommain
tofeature-auth
. - Merging Branches: Use
git merge <branch_name>
to integrate changes from one branch into another. If you developed a specific feature onfeature-auth
, you can merge it back intomain
. - Deleting Branches: After completing a branch, delete it with
git branch -d <branch_name>
locally orgit push origin --delete <branch_name>
for remote removal.
- Use Descriptive Names: Ensure branch names reflect their purpose (e.g.,
hotfix/payment-issue
). - Avoid Long-Lived Branches: Regularly integrate changes into the main branch to reduce conflicts.
- Check Outdated Branches: Periodically review for unused branches to keep the repository clean.
Understanding these core concepts ensures effective collaboration and seamless code integration within your projects.
Why Rename A Git Branch?
Renaming a Git branch enhances clarity, avoids confusion, and improves collaboration. It ensures branch names reflect their purpose accurately, aligning with best practices for organized version control.
Common Scenarios For Renaming Branches
- Correcting Typographical Errors: Mistyped branch names, such as
feture/add-login
instead offeature/add-login
, hinder searchability and understanding. - Adjusting Naming Conventions: Teams often standardize branch naming conventions, for example, switching from camelCase to kebab-case (
featureAddLogin
tofeature-add-login
) to maintain consistency. - Repurposing Branches: When a branch’s purpose evolves, such as extending a feature branch to a larger project, renaming communicates the change.
- Avoiding Ambiguity: Names like
test
orupdate
lack context. More descriptive names, liketesting-db-migrations
, prevent miscommunication.
- Improved Collaboration: Descriptive, standardized branch names help team members understand each branch’s role without extensive explanation.
- Enhanced Repository Organization: Clear, consistent branch names denote development stages, planned features, or fixes, streamlining code base navigation.
- Reduced Errors: Accurate branch names avoid accidental merges, pull requests on incorrect branches, or redundant development efforts.
- Better Reflects Project Scope: Renaming ensures that branch names align with changes in scope, reducing misunderstandings around active development.
Steps To Rename A Git Branch
Renaming a Git branch involves a series of straightforward steps. This section explains how to rename both local and remote branches while maintaining consistency and organization within your repository.
Renaming A Local Branch
Follow these steps to rename a branch locally:
- Switch From the Target Branch
Ensure you’re not currently on the branch you intend to rename. Use the git switch
command to move to another branch:
git switch <branch-name>
- Rename the Branch Locally
Use the git branch -m
command to rename the branch:
git branch -m <old-branch-name> <new-branch-name>
If you’re on the branch being renamed, leave out the <old-branch-name>
argument:
git branch -m <new-branch-name>
- Verify the Local Renaming
Confirm the branch has been renamed by listing all local branches:
git branch
These steps rename the branch locally but don’t update the remote repository, which is covered in the next section.
Renaming A Remote Branch
After renaming a local branch, follow these steps to reflect the changes on the remote repository:
- Delete the Old Remote Branch
Remove the outdated branch from the remote by running:
git push origin --delete <old-branch-name>
- Push the Renamed Branch
Add the renamed branch to the remote repository using:
git push origin <new-branch-name>
- Update Tracking Information
Set up tracking for the new branch by linking it to the remote branch:
git branch --set-upstream-to=origin/<new-branch-name>
- Confirm Remote Changes
Verify the renaming by listing remote branches with:
git branch -r
This process ensures your remote repository is aligned with your local changes, promoting cleaner collaboration and reduced errors.
Best Practices For Renaming Git Branches
Renaming Git branches enhances collaboration and ensures your repository stays organized. Following best practices reduces errors and streamlines the renaming process.
Communication With Team Members
Inform your team before renaming a branch to avoid confusion. Notify them about the new branch name and the reason for renaming via emails, collaboration tools, or version control platform comments. This ensures everyone is aware of updates.
Update documentation if applicable to reflect the new branch name. For example, modify README files, wikis, or internal project notes referencing the old name to prevent outdated information.
Encourage your team to update their local repositories after renaming. They can do this by fetching the latest changes and checking out the renamed branch using its updated name.
Avoiding Conflicts During Renaming
Ensure no pending changes exist in the branch before renaming. Stash or commit any uncommitted changes to prevent loss of work or merge conflicts.
Coordinate renaming during low activity periods in the repository. Overlap between concurrent edits and the renaming process increases the risk of conflicts.
Delete the old remote branch only after successfully pushing the renamed branch. Then update tracking information for the new branch using these commands:
git branch --unset-upstream
git push -u origin <new-branch-name>
Verify the new branch’s integration to confirm it aligns with all ongoing workflows and tools linked to your repository.
Common Errors And Troubleshooting
Renaming a Git branch can occasionally lead to unexpected errors, especially when managing remote repositories or dealing with merge conflicts. Addressing these issues promptly ensures smooth project workflows.
Issues With Remote Repositories
Errors with remote repositories often arise when renaming a branch without properly updating the remote repository. For instance, failing to delete the old branch or push the renamed branch can create discrepancies.
- Unable To Push Renamed Branch: If the new branch name isn’t pushed to the remote, Git rejects the push. Use
git push origin <new-branch>
to upload the renamed branch. Confirm the push by runninggit branch -r
. - Facing Tracking Issues: After renaming locally, the old branch may still track its remote counterpart. Run
git branch --unset-upstream
followed bygit branch -u origin/<new-branch>
to update the branch tracking information. - Old Branch Still Visible: A deleted branch might still appear in remote listings if it’s not explicitly removed. Use
git push origin --delete <old-branch>
to clean up the remote repository.
Resolving Merge Conflicts
Merge conflicts can occur during branch renaming, typically when uncommitted changes are present or the branches aren’t synchronized.
- Uncommitted Changes Blocking Rename: Git prevents renaming a branch with pending changes. Commit or stash changes using
git commit -m "message"
orgit stash
, then proceed with renaming. - Conflict After Pushing Renamed Branch: If the renamed branch conflicts with an existing branch, Git flags it. Rebase the branch onto the latest commits using
git rebase origin/main
, resolve conflicts in the affected files, and complete the rebase withgit rebase --continue
. - Issues During Pull Requests (PRs): Renaming branches linked to open PRs can break the PR. Update the PR’s base branch in the repository’s interface, or re-create the PR using the new branch name.
Addressing these common errors ensures seamless integration of renamed branches into your Git workflow, minimizing potential disruptions.
Tools And Resources For Efficient Git Branch Management
Efficient Git branch management relies on selecting the right tools and resources for version control, collaboration, and workflow optimization.
1. Git Command Line Interface (CLI)
The Git CLI offers direct access to Git’s functionality. Use commands like git branch
, git checkout
, and git merge
to create, switch, or manage branches. It’s an essential tool for those who prefer full control and flexibility within their workflows.
2. Integrated Development Environments (IDEs)
IDEs like Visual Studio Code, IntelliJ IDEA, and PyCharm provide built-in Git support. Use their GUI features to visualize branch structures, resolve merge conflicts, and perform Git actions without relying on command-line input.
3. Version Control Systems (VCS) Platforms
Platforms like GitHub, GitLab, and Bitbucket enhance Git functionality. Use their web interfaces to manage branches, review pull requests, and integrate CI/CD workflows. Features like branch protection rules, status checks, and review assignments simplify collaborative coding.
4. Branch Management Tools
Tools like Sourcetree and Fork present a graphical overview of your Git repository. Use them to track changes, visualize commit history, and simplify Git operations. These tools are valuable for users who prefer GUI-based branch management.
5. Documentation And Guides
Git’s official documentation, tutorials, and community forums help resolve specific issues. Refer to resources like Git-scm.com and Stack Overflow to understand Git commands, troubleshoot errors, and adopt best practices for branch naming and management.
6. Git Extensions And Plugins
Enhance IDEs and editors with Git-specific extensions. For example, Visual Studio Code’s GitLens provides insights into commit histories and authorship while improving branch and stash access. Explore IDE-specific marketplaces for compatible plugins.
7. Collaboration Tools
Integrate communication platforms like Slack or Microsoft Teams with Git to streamline notifications about branch changes. Use these tools for team discussions, deployment updates, and quick resolution of Git-related queries.
8. Code Review And Analysis Tools
Tools like Crucible and SonarQube assist in branch code quality analysis. Use them to detect bugs, enforce standards, and review code changes during pull requests, ensuring higher-quality contributions across branches.
9. Continuous Integration/Continuous Deployment (CI/CD)
CI/CD tools like Jenkins, CircleCI, and Travis CI automate tasks such as testing and deployment. Integrate them with branches to maintain stable code by automatically pulling, testing, and deploying changes.
Use the above resources to maintain clean and organized repositories, foster team collaboration, and improve your overall development workflows.
Conclusion
Renaming a Git branch is a valuable skill that helps keep your repository organized and your workflows efficient. By following the outlined steps and adopting best practices, you can ensure smooth collaboration and avoid common pitfalls.
Whether you’re correcting a typo or aligning branch names with project goals, clear communication and proper execution are key. With the right tools and resources, you can confidently manage branches and maintain a streamlined development process.
Frequently Asked Questions
Why would I need to rename a Git branch?
Renaming a Git branch is often necessary to improve clarity, correct typographical errors, align with naming conventions, repurpose an existing branch, or reduce ambiguity. Descriptive branch names enhance collaboration and ensure better organization within a repository.
How do I rename a local Git branch?
To rename a local branch, switch off the branch you want to rename, then use the git branch -m old-name new-name
command. Confirm the changes by listing local branches with git branch
afterward.
How can I rename a remote Git branch?
To rename a remote branch, delete the old branch on the remote with git push origin --delete old-name
, push the renamed branch using git push -u origin new-name
, and update tracking information with git branch --set-upstream-to=origin/new-name
.
What should I do before renaming a Git branch?
Ensure no pending changes exist on the branch, notify team members about the renaming in advance, and plan the process during a low activity period to avoid conflicts. It’s also helpful to update relevant documentation.
What are some best practices for renaming Git branches?
Use descriptive names, communicate changes with your team, and regularly review branches to avoid long-lived or outdated branches. Keep your repository clean and aligned with the project’s goals.
How do I resolve errors when renaming a Git branch?
If issues arise, such as failing to push the renamed branch or lingering old branches in remote listings, revisit the steps for removing or pushing branches. For tracking issues, update branch tracking with git branch --set-upstream-to=origin/new-name
.
What are Git branches used for?
Git branches provide independent environments for experimentation, feature development, and bug fixing, all without affecting the main project. They streamline version control by isolating specific tasks.
How can tools help with Git branch renaming and management?
Tools like Git CLI, IDEs, GitHub, or GitLab can visualize branch processes, manage workflows, and ensure efficient collaboration. Additional tools like CI/CD systems and code review tools enhance project organization and productivity.
Can I rename a branch with uncommitted changes?
It’s not recommended to rename a branch with uncommitted changes as this can lead to conflicts. Commit or stash your changes before renaming to ensure a smooth process.
Does renaming a branch affect team workflows?
Renaming can impact teams if not communicated effectively. Notify team members, update tracking information, and ensure everyone aligns with the new branch name to maintain seamless collaboration.